Introduction ( Python tips for beginners )
Learning Python can be a fun and rewarding journey, especially with the right tips and techniques to guide you. As one of the most popular programming languages in the world, Python is known for its simplicity and versatility, making it a great choice for beginners. Whether you’re just starting out or looking to improve your skills, these Python tips for beginners will help you write cleaner, more efficient code.
In this article, we’ll explore 10 essential tips that every beginner Python programmer should know to boost their coding skills. By the end, you’ll have a solid foundation to write better Python code and avoid common mistakes.
Table of Contents
1. Understand Python’s Syntax and Structure
One of the first things you’ll notice about Python is its clean and readable syntax. Python uses indentation to define the structure of the code, which eliminates the need for curly braces {}
or semicolons ;
used in other programming languages.
Example:

In this example, the indentation signifies that print()
is part of the greet
function. Always maintain consistent indentation (4 spaces) to avoid syntax errors.
2. Use Meaningful Variable Names
Using clear, descriptive variable names makes your code more readable and easier to maintain. Avoid single-letter or cryptic names, and instead use names that describe the purpose of the variable.
Example:
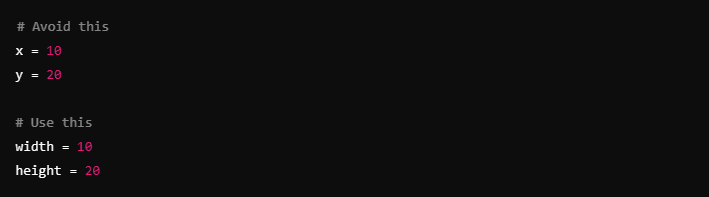
Good variable names will make your code easier for others to understand and help you when you revisit the code later.
3. Leverage Python’s Built-In Functions
Python comes with a variety of built-in functions that can simplify your code and reduce redundancy. Functions like len()
, sum()
, max()
, and min()
are commonly used to perform operations on lists, strings, and other data structures.
Example:

This code sums up the numbers in the list without having to write a loop. Make sure to familiarize yourself with Python’s built-in functions to save time.
4. Master List Comprehensions
List comprehensions allow you to create lists in a more concise and readable way. Instead of writing loops to generate or modify lists, you can use list comprehensions to achieve the same result with less code.
Example:
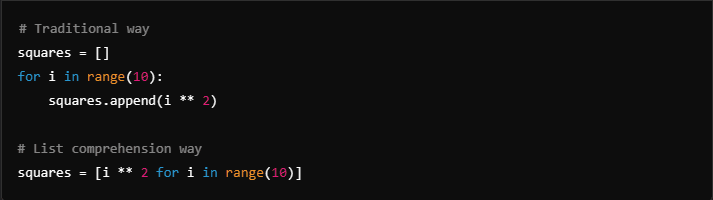
Using list comprehensions can make your code cleaner and more Pythonic, especially when working with lists.
5. Get Comfortable with Python’s Data Structures
Python offers a variety of built-in data structures, such as lists, tuples, sets, and dictionaries. Understanding when and how to use each of these data structures is crucial for writing efficient Python code.
- Lists: Ordered, mutable collections.
- Tuples: Ordered, immutable collections.
- Sets: Unordered collections of unique items.
- Dictionaries: Key-value pairs for fast lookups.
Example:

Dictionaries are especially useful when you need to store data that can be quickly accessed by keys.
6. Handle Exceptions Gracefully
Errors and exceptions are inevitable when programming, but Python provides a way to handle these errors gracefully using try
, except
, and finally
blocks. This helps prevent your program from crashing and allows you to manage errors in a controlled manner.
Example:
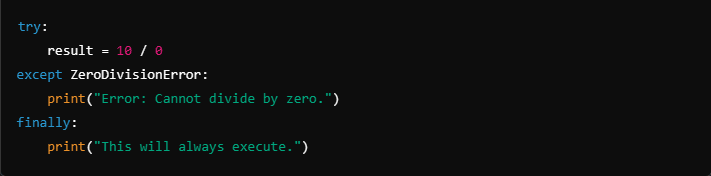
Handling exceptions properly can make your code more robust and user-friendly.
7. Use Virtual Environments for Project Isolation
When working on multiple Python projects, it’s important to isolate dependencies to avoid conflicts between packages. Python’s venv
module allows you to create virtual environments for each project, ensuring that each project has its own set of dependencies.
Example:
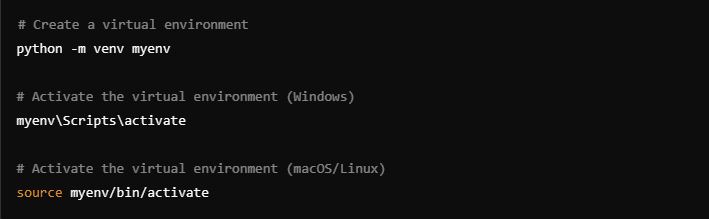
Using virtual environments keeps your projects organized and prevents dependency issues.
8. Optimize Your Code with Python Libraries
Python has a vast ecosystem of libraries that can help you perform tasks more efficiently. Whether you’re working with data, web development, or automation, there’s likely a library that can help you achieve your goals faster.
Popular Python Libraries:
- NumPy: For numerical computing.
- Pandas: For data manipulation.
- Flask/Django: For web development.
- Requests: For making HTTP requests.
Using the right libraries can significantly reduce the amount of code you need to write and improve your code’s performance.
9. Write Readable and Maintainable Code
Writing readable code is just as important as writing code that works. Python emphasizes readability and clarity, so take the time to write code that others can easily understand.
Best Practices:
- Use meaningful variable and function names.
- Keep functions small and focused on a single task.
- Write comments to explain complex logic.
- Follow PEP 8 guidelines, Python’s style guide.
Readable code is easier to maintain and debug, which will save you time in the long run.
10. Keep Learning and Experimenting with Python
Python is a language that evolves over time, and new features, libraries, and best practices are constantly being developed. To stay ahead, keep learning and experimenting with Python. Try building small projects, contributing to open-source, or exploring new libraries and frameworks.
Conclusion
By applying these Python tips for beginners, you’ll be able to write cleaner, more efficient, and more maintainable code. From understanding Python’s syntax and structure to using libraries and virtual environments, these tips will help you take your Python skills to the next level.
Remember, the key to mastering Python (or any programming language) is practice. Keep experimenting with different techniques and projects, and you’ll continue to improve over time.
Previous Python Article