Introduction
Object-oriented programming (OOP) in Python is a fundamental concept that every developer should master to write clean, efficient, and scalable code. Python’s OOP model simplifies the structure of programs by organizing code into reusable and modular components, which makes it easier to manage and extend applications. OOP principles are used across various domains, from game development to web applications and machine learning models.
In this article, we’ll dive deep into the seven key concepts of Object-Oriented Programming in Python, explaining each concept with examples to help you understand how to apply OOP effectively in your projects.
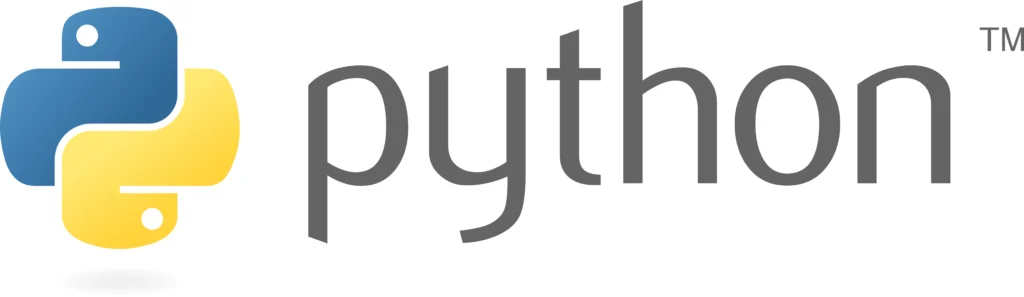
Table of Contents
1. What is Object-Oriented Programming in Python?
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of objects, which can contain data (attributes) and functions (methods). In Python, everything is an object, making it a perfect language to explore OOP principles.
Using OOP, developers can break down complex programs into smaller, more manageable pieces, making the code more organized and modular. The four main principles of OOP are encapsulation, inheritance, polymorphism, and abstraction, which we’ll explore in this guide.
2. Classes and Objects: The Building Blocks of OOP
In Python, classes are templates or blueprints that define the properties and behaviors of an object. Objects are instances of classes, and they carry the properties and behaviors defined by their respective class.
Example of a Class and Object:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def drive(self):
return f"The {self.brand} {self.model} is driving."
# Creating an object (instance) of the Car class
my_car = Car("Toyota", "Corolla")
print(my_car.drive())
In this example, the class Car
defines a blueprint for a car with attributes like brand
and model
. The object my_car
is an instance of the Car
class, and it can use the drive
method defined in the class.
Key Takeaway: Classes define properties and behaviors, while objects are individual instances of these classes that use the properties and behaviors.
3. Encapsulation: Hiding Data for Security
Encapsulation is the process of bundling data (attributes) and methods that operate on the data within a class and restricting access to certain components. It allows for hiding the internal state of an object and only exposing a controlled interface for interaction.
Python uses single and double underscores to indicate protected and private members, respectively:
-
_variable
: Protected (can be accessed but should not be). __variable
: Private (not accessible outside the class).
Example of Encapsulation:
class Employee:
def __init__(self, name, salary):
self.name = name # Public attribute
self.__salary = salary # Private attribute
def get_salary(self):
return self.__salary
employee = Employee("John", 50000)
print(employee.name) # Accessible
# print(employee.__salary) # Not accessible (will raise an AttributeError)
print(employee.get_salary()) # Accessible via method
Encapsulation ensures that the class controls how its data is accessed and modified, which enhances security and code maintainability.
4. Inheritance: Reusing Code Across Classes
Inheritance is a powerful feature of OOP that allows one class (child or subclass) to inherit the attributes and methods of another class (parent or superclass). This promotes code reusability and can help reduce redundancy.
Example of Inheritance:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return f"{self.name} makes a sound."
class Dog(Animal):
def speak(self):
return f"{self.name} barks."
my_dog = Dog("Rex")
print(my_dog.speak())
In this example, the Dog
class inherits from the Animal
class and overrides the speak
method to provide its specific implementation.
Key Takeaway: Inheritance allows you to create a hierarchy of classes where subclasses inherit and extend the behavior of parent classes.
5. Polymorphism: Flexibility in Code
Polymorphism allows different objects to be accessed through the same interface, enabling functions or methods to operate on objects of various types. In Python, polymorphism can be achieved by method overriding and operator overloading.
Example of Polymorphism:
class Bird:
def sound(self):
return "Chirp"
class Dog:
def sound(self):
return "Bark"
def make_sound(animal):
print(animal.sound())
my_bird = Bird()
my_dog = Dog()
make_sound(my_bird)
make_sound(my_dog)
In this example, both Bird
and Dog
classes implement a sound
method, and the make_sound
function can handle both objects, demonstrating polymorphism.
6. Abstraction: Simplifying Complex Systems
Abstraction involves hiding the complex implementation details of a system and exposing only the essential parts. This helps reduce complexity and increases the usability of the system by focusing on what it does rather than how it works.
In Python, abstraction is often achieved using abstract base classes (ABCs). ABCs define methods that subclasses must implement.
Example of Abstraction:
from abc import ABC, abstractmethod
class Vehicle(ABC):
@abstractmethod
def drive(self):
pass
class Car(Vehicle):
def drive(self):
return "Car is driving."
my_car = Car()
print(my_car.drive())
The Vehicle
class is an abstract class with an abstract method drive
. Any subclass like Car
must implement the drive
method.
7. Composition vs. Inheritance
In OOP, composition is another way to create relationships between objects. Rather than using inheritance, composition involves including one class as a part of another class.
Example of Composition:
class Engine:
def start(self):
return "Engine started."
class Car:
def __init__(self):
self.engine = Engine() # Composition
def start_car(self):
return self.engine.start()
my_car = Car()
print(my_car.start_car())
In this example, the Car
class contains an Engine
object as part of its properties. This approach is often preferred over inheritance for flexibility and modularity.
Key Takeaway: Use composition when a class should include another class, and use inheritance when a class should inherit behavior from a parent class.
8. Conclusion
Mastering Object-Oriented Programming in Python allows you to write more efficient, modular, and scalable code. The key OOP concepts—classes, encapsulation, inheritance, polymorphism, abstraction, and composition—empower you to design applications that are easy to maintain and extend.
By applying these concepts, you can build complex systems while keeping your code organized and understandable. Keep practicing these principles in your Python projects, and you’ll soon see improvements in the quality and structure of your code.
Previous Python Article
5 Powerful Ways to Use Python for Automation: Simplify Your Daily Tasks